Master Python Match Group for Effective Pattern Matching
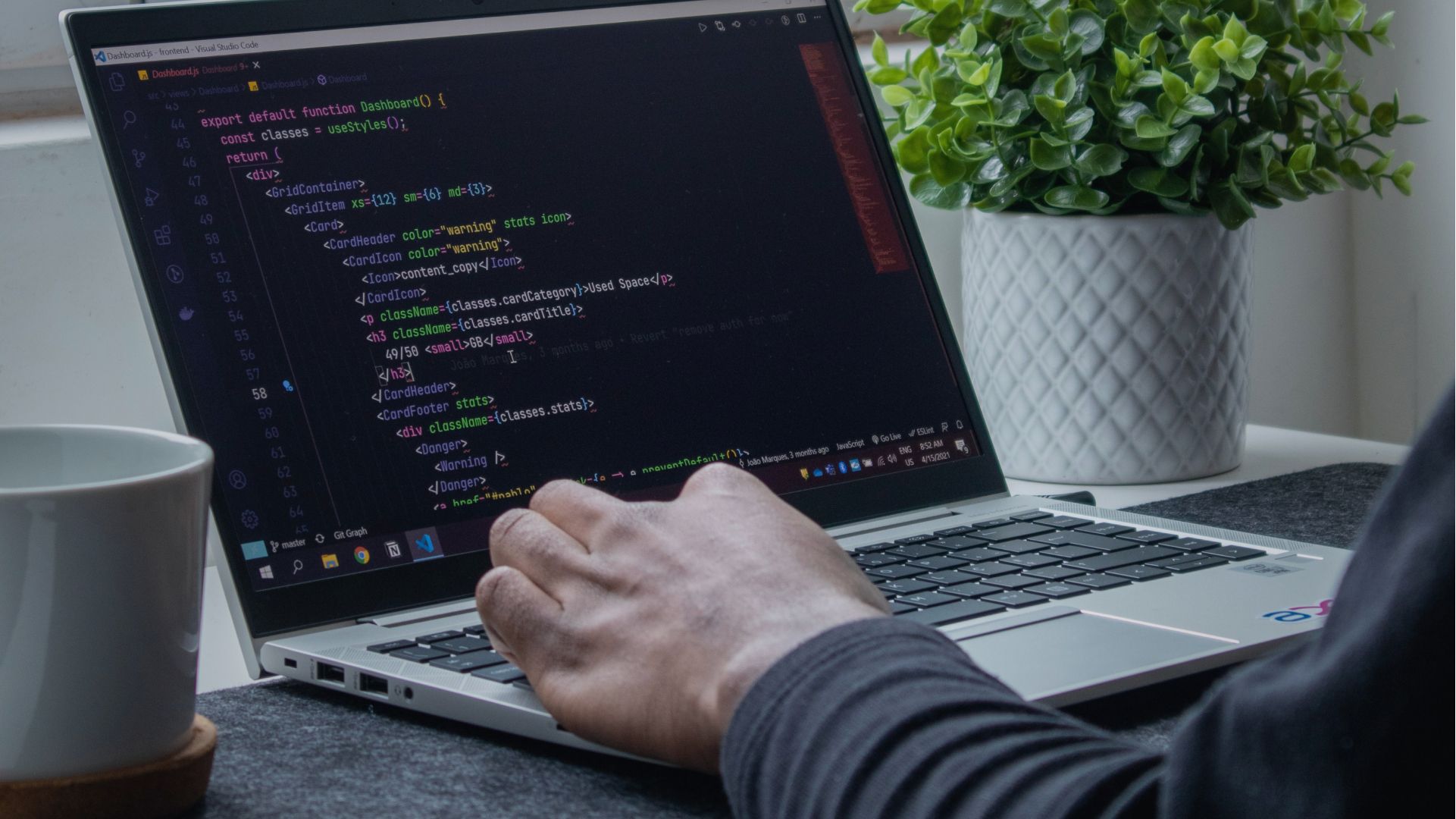
In the world of programming, pattern matching is a powerful technique that allows developers to compare complex data structures against specific patterns, making code more readable, concise, and efficient. Python, a language renowned for its simplicity and versatility, introduced the match statement (also known as the match group) in Python 3.10, bringing pattern matching capabilities to the language. This feature, inspired by functional programming languages like Haskell and ML, enables developers to handle data in a more intuitive and structured way. In this article, we’ll explore the Python match group, its syntax, use cases, and best practices to help you master this powerful tool.
Understanding Python’s Match Statement
The match
statement is Python’s answer to pattern matching, providing a way to compare a value against a series of patterns and execute code based on the first successful match. It’s particularly useful for handling complex data structures like dictionaries, lists, and custom objects.
Basic Syntax
match subject:
case pattern_1:
# Code to execute if pattern_1 matches
case pattern_2:
# Code to execute if pattern_2 matches
case _:
# Default case (wildcard)
Here, subject
is the value being matched, and pattern_
represents the structure you’re looking for.
Key Features of Python Pattern Matching
1. Matching Literals
You can match against simple literals like strings, numbers, or None
.
def process_status(status):
match status:
case "success":
return "Operation completed successfully."
case "error":
return "An error occurred."
case _:
return "Unknown status."
2. Matching Sequences
Patterns can include sequences like lists or tuples, allowing you to extract elements.
def describe_point(point):
match point:
case (0, 0):
return "Origin"
case (x, 0):
return f"On the X-axis at {x}"
case (0, y):
return f"On the Y-axis at {y}"
case (x, y):
return f"Point at ({x}, {y})"
3. Matching Dictionaries
Dictionary patterns allow you to extract keys and values.
def process_user(user):
match user:
case {"name": name, "age": age}:
return f"{name} is {age} years old."
case {"name": name}:
return f"Hello, {name}!"
case _:
return "Unknown user format."
4. Using Guards (Conditional Matching)
Add conditions to patterns using if
clauses.
def categorize_number(num):
match num:
case n if n > 0:
return "Positive"
case 0:
return "Zero"
case n if n < 0:
return "Negative"
5. Capturing Sub-Patterns
Extract values from nested structures.
def process_data(data):
match data:
case {"type": "user", "id": id, "name": name}:
return f"User {id}: {name}"
case {"type": "event", "name": event_name}:
return f"Event: {event_name}"
case _:
return "Unknown data format."
6. Wildcard Pattern (_
)
The wildcard matches anything and is often used as a default case.
def check_value(value):
match value:
case 42:
return "The answer to life!"
case _:
return "Just a regular value."
Practical Use Cases
1. Data Validation
Pattern matching simplifies validating complex data structures.
def validate_config(config):
match config:
case {"host": str(), "port": int()}:
return "Valid configuration."
case _:
return "Invalid configuration."
2. Parsing JSON Data
Handle JSON responses with ease.
import json
def parse_response(response):
data = json.loads(response)
match data:
case {"status": "success", "data": [{"id": id, "name": name}]}:
return f"ID: {id}, Name: {name}"
case {"status": "error", "message": msg}:
return f"Error: {msg}"
case _:
return "Unknown response format."
3. State Machines
Implement state machines with clear, readable code.
def handle_state(state):
match state:
case "start":
return "Initialize system."
case "running":
return "System is operational."
case "error":
return "System error detected."
case "stop":
return "System shutdown."
case _:
return "Unknown state."
Best Practices for Using Match Groups
- Keep Patterns Simple: Avoid overly complex patterns. If a pattern becomes too intricate, consider refactoring.
- Use Guards Sparingly: While guards are powerful, overuse can make code harder to read.
- Leverage the Wildcard: Always include a wildcard case to handle unexpected inputs gracefully.
- Combine with Type Hinting: Use type hints to make patterns more explicit and improve code clarity.
- Test Thoroughly: Pattern matching relies on the order of cases. Test all possible inputs to ensure correctness.
Comparing match
vs. if-elif-else
While if-elif-else
chains can achieve similar results, match
is more concise and readable for complex pattern matching.
Example with if-elif-else
:
def check_number(num):
if num > 0:
return "Positive"
elif num == 0:
return "Zero"
else:
return "Negative"
Equivalent with match
:
def check_number(num):
match num:
case n if n > 0:
return "Positive"
case 0:
return "Zero"
case n if n < 0:
return "Negative"
Future of Pattern Matching in Python
The match
statement is still evolving, and future Python releases may introduce additional features, such as:
- Custom pattern matching protocols for user-defined classes.
- Enhanced sequence matching for more complex data structures.
- Integration with third-party libraries for advanced use cases.
When should I use `match` instead of `if-elif-else`?
+Use `match` when dealing with complex data structures or multiple conditions that check against specific patterns. It’s more readable and concise for such cases.
Can I use `match` with custom objects?
+Currently, `match` works best with built-in types like lists, tuples, and dictionaries. Support for custom objects may improve in future Python releases.
Is `match` slower than `if-elif-else`?
+`match` is optimized for performance and is generally as fast as equivalent `if-elif-else` chains. However, complexity can impact speed, so test for your specific use case.
How does `match` handle nested patterns?
+`match` supports nested patterns, allowing you to extract values from deeply nested structures. For example, `{"user": {"name": name}}` can capture the `name` field.
Can I use `match` in Python versions before 3.10?
+No, the `match` statement was introduced in Python 3.10. For earlier versions, you’ll need to use `if-elif-else` or third-party libraries like `matchpy`.
By embracing Python’s match group, you’ll unlock new levels of efficiency and clarity in your code. Whether you’re parsing data, validating inputs, or implementing complex logic, pattern matching is a skill every Python developer should master. Happy coding!